CSCI 150: PreLab 7
Recursion
Due:
9AM
on
Wednesday,
October 30th
In this prelab you will formulate some of the ideas necessary to complete Lab 06. Please turn in your solution on Gradescope. You can either turn it in as a PDF (e.g., saving a document as a PDF in Word), take a picture (e.g., with a smartphone), or scan it (e.g., at the library) to hand it in. Please remember, no late prelabs allowed!
Reading
Read this article about Computer Science in everyday life.
Recursion with Numbers
As we have seen (or will have seen, depending on when you're looking at this), the factorial function can be computed not only using a loop, but via recursion as well. Recall the the typical definition of factorial looks something like this: 0! = 1, 1! = 1, 2! = 2*1 = 2 and 3! = 3*2*1 = 6, and in general, n! = n*(n-1)*...*3*2*1.
But we could also define the factorial function recursively as follows. We'll use fact(n) to denote our recursive representation of n!. We'll define fact(n) = 1 when n is 0 and fact(n) = n*fact(n-1) otherwise. If you think about it, fact(n) gives the exact same values as n!.
3. Give an analogous recursive definition for the sum of the first n perfect squares. For example, your definition should satisfy sps(1) = 1, sps(2) = 1 + 4 = 5, and sps(3) = 1 + 4 + 9 = 14.
Recursion with Strings
Here is pseudocode for recursively printing a string s in reverse
If the length of s is 0, do nothing Otherwise, print the last letter of s and reverse s with the last letter removed.
Fractal Images
Consider the following figure.
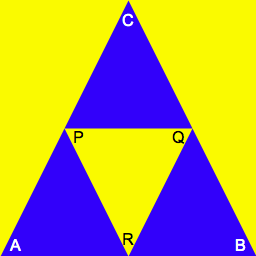
Let Ax and Ay denote the x and y coordinates of the corner labelled A in the large triangle above. Let Bx, By, Cx and Cy be definied similarly. Assume P, Q and R each lie at the midpoint of their corresponding edge in the triangle ABC.
Honor Code
If you followed the Honor Code in this assignment, write the following sentence attesting to the fact at the top of your homework.
I affirm that I have adhered to the Honor Code in this assignment.