CSCI 364: Homework Assignment #1
Search for a Restaurant!
Due: 2:30 PM on Wednesday, October 27 11:59 PM on Friday, October 29
You can download the assignment instructions by clicking on this link
Code Update! Click here to download faster versions of map.py and Map.java that will drastically speed up how fast they read in the map files (eliminating the long load times in the versions of these files up on GitHub).
Instructions for using GitHub for our assignments can be found on the Resources page of the class website, as well as using this link.
Testing Your Code
In your assignment's Maps folder, I've included a small test file called mapT.dat that encodes the following map to help you hand trace your code and debug:
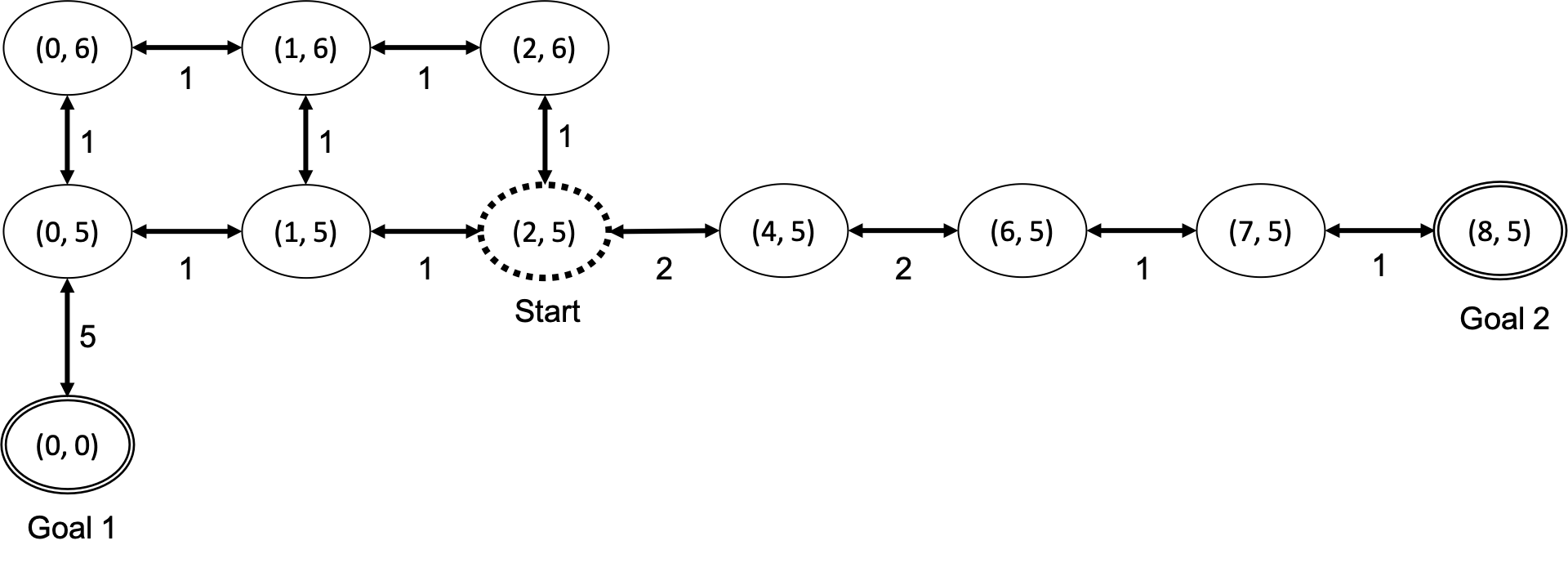
The outputs you calculate should resemble the following:
BFS Path Length: 3 BFS Path Cost: 7.0 BFS Goal Reached: (0.0, 0.0) Nodes Expanded with BFS: 5 Time Spent with BFS: 7.700920104980469e-05 UCS Path Length: 4 UCS Path Cost: 6.0 UCS Goal Reached: (8.0, 5.0) Nodes Expanded with UCS: 9 Time Spent with UCS: 0.0002579689025878906 A* Path Length: 4 A* Path Cost: 6.0 A* Goal Reached: (8.0, 5.0) Nodes Expanded with A*: 4 Time Spent with A*: 0.00019407272338867188
Reading in a Map File
To read in a map file in Python and turn it into an instance of the Problem class, you will want to use the following code:
import map
import problem
my_map = map.readFromFile(filename)
problem_model = problem.Problem(my_map)
and in Java:
Map map = Map.readFromFile(filename);
Problem problemModel = new Problem(map);
Timing Operations
To calculate how long something takes in Python, we use time.process_time() from the time module. A short example program is:
import time
startTime = time.process_time()
print("How long does it take to call a print statement?")
endTime = time.process_time()
duration = endTime - startTime
print("Answer:", duration, "seconds")
In Java, we use System.currentTimeMillis(). The same short example program is:
long startTime = System.currentTimeMillis();
System.out.println("How long does it take to call a print statement?");
long endTime = System.currentTimeMillis();
double duration = (endTime - startTime) / 1000; # convert to seconds
System.out.println("Answer: " + duration + " seconds");